Selection Sort
Basically in this technique we take minimum element from entire array & swap it with the 1st element. After this we take minimum from entire array excluding 1st element & swap that minimum element & element at 2nd position & continue so on.
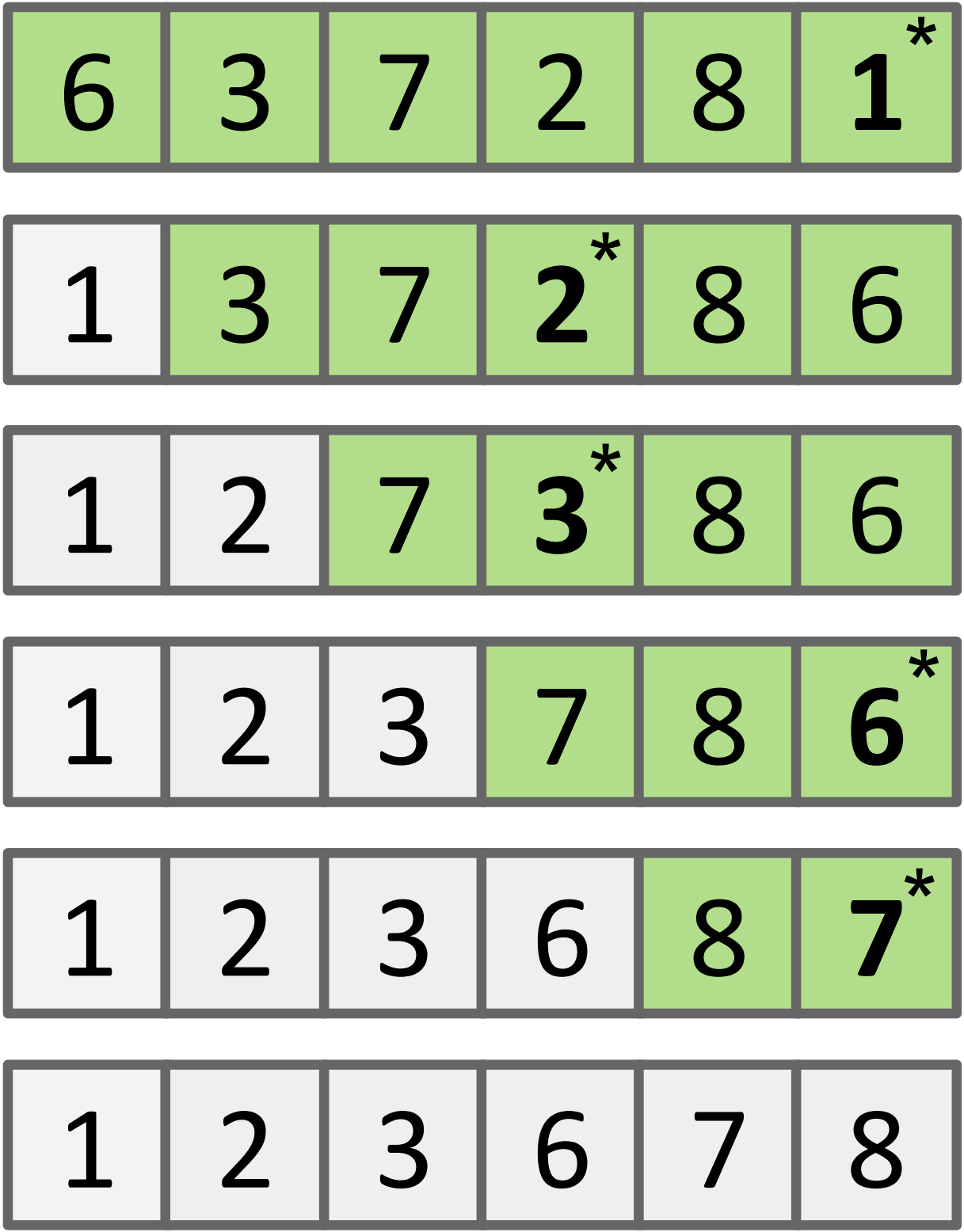
ALGORITHM :
FOR i = 1 TO N - 1 DO min = i FOR j = i + 1 TO N DO IF A[j] < A[min] THEN min = j SWAP(A[min], A[i])
Source Code :
void *sort(int a[], int len) { int i, j, min, tmp, pos; for(i = 0; i<len-1; i++) { min = i; for(j = i+1; j<len; j++) { if(a[j] < a[min]) min = j; } tmp = a[min]; a[min] = a[i]; a[i] = tmp; } return a; }
No comments:
If you have any doubt or suggestion let me know in comment section.