CRUD (Create, Read, Update, Delete) using Node.js & MongoDB
Prerequisites : Node.js & MongoDB
Full Source Code available at : GitHub
Packages Used :
Project Setup : Create a folder with any name and git bash in it. Now follow up the command to download different packages for the project.
Create package.json :
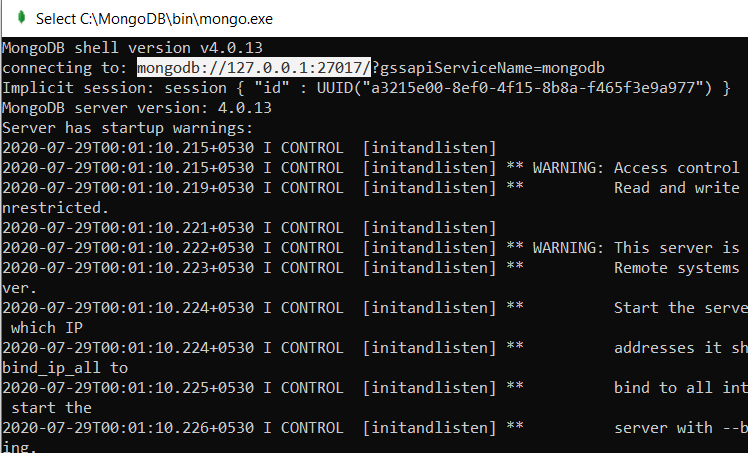
Now we will create our default routes.
1234567app.all('/', function(req, res){ return res.json({ staus: true, message : 'Index page is working' }); });
123456789101112131415161718192021222324// init code const mongoose = require('mongoose'); const assert = require('assert'); const db_url = process.env.DB_URL; // connection code mongoose.connect( db_url, { useNewUrlParser : true, useUnifiedTopology : true, useCreateIndex : true }, // link stores connection of database function(error, link){ // check error assert.equal(error, null, 'DB Connect fail'); // OK console.log("DB Connect Success"); console.log(link); } );
it
into a
Model we can work with.
1234567891011121314151617181920212223const userSchema = mongoose.Schema({ username : { type : String, required : true }, email : { type : String, required : true }, password : { type : String, required : true }, isActive : { type : Boolean, default : true }, createdOn : { type : Date, default : Date.now() } });
Middle ware :
Now when you will visit http://localhost:3000/api/user/ you will notice below display which is coming from controllers folder:
Now we will see how we can use express validator to validitate & sanitize the HTTP requests coming to server side from form.
First we will create route 'createNew' in controller. Then we will check whether the username is empty or not & then trim the username using .trim() (trim removes whitespaces present before & after the username). At last we will use .escape() (converts html special sysmbols into corresponding html code)
Then will follow same for password & email. For email we use isEmail() (built-in function that checks the validity of given email), normalizeEmail() (sanitize the email).
Then we will create function which check for error & in case of error return 422 status code & pass json file showing validitation error. Else we will simply return json file showing no error foumd & user data.
After this using bcryptjs to hash our password.
Now we will learn how to save our data in our database. For this we will use create() function & save username & password in database.
Since our password is hashed we will use the variable in which we have stored hashed password in our case its hashedPassword.
Now we will see how we can use create() function to store details in database.
12345678910111213User.create( { username : req.body.username, email : req.body.email, password : hashedPassword }, function(error, result){ // check error if (error) res.send("Error"); else res.send("Successful"); } );
But we will not use this code instead we will use save() function to save data into database.
1234567891011var temp = new User({ username : req.body.username, email : req.body.email, password : hashedPassword }); temp.save(function(error, result){ if (error) res.send("Error"); else res.send("Successful"); });
Now to check working of this route open postman & go to URL localhost:3000/api/user/createNew
Now we will see how we can read data from our mongoDB database using /find route. For this we will use our 'User' model & find() method to find the data present in database. And then simply check for error else return the data. Now just run js file & your mongoDB server & from the browser go to :
Now we have covered Create & Read. Next is Update route in this put() method will be used. In this we have to update the username of email passed with the URL /update/:email.
First we will check whether the email is matching with the email present in our database by req.params.email If email matches then we will define what all things to update. In our case we are updating the username. And then simply check for error else return that everything is okay. Now since everything is done. Open your postman & pass email in the URL just as done below :
Now in browser open http://localhost:3000/api/user/find you will find username updated to Shubham Arora.
Moving ahead to delete route. Just as update route we will pass email on our URL whose data we want to delete (localhost:3000/api/user/delete/milint@gmail.com)
Now on browser http://localhost:3000/api/user/find you will not find the record associated with this email.Since we have completed all our CRUD task now we will see about login route & also how we can match our hashed password.
Important note : Throughout this tutorial we haven't used html form for sending data instead we have used postman to send data to server-side.
Moving ahead to login route; first we will validate our data using html validator
Then we will check email exists or not in our database by this code : { email : req.body.email }. Finally we check check for error & check result matches or not. If result doesn't match we simply return error. Now using bcryptjs we check the password entered by user (req.body.password) with the password present in our database in hashed form (result.password).
At last simply check password is matching or not using isMatch. Now simply go to postman at localhost:3000/api/user/login URL. Then just pass email & password you will get results accordingly.
Video ref. Sandeep Ganguli
No comments:
If you have any doubt or suggestion let me know in comment section.